How to Set a Unique Input Column Using Filament & Laravel 11
When building a web application with Laravel 11 and Filament, you might encounter a situation where you need to ensure a specific input field, such as an email, remains unique during the creation or editing of records. Fortunately, Filament provides an elegant solution to handle this with its TextInput
component.
This tutorial will walk you through how to make an input field unique, ensuring that validation rules work seamlessly whether you're creating or editing a record.
Prerequisites
Before diving into the code, make sure you have the following:
-
Laravel 11 installed and set up in your project.
-
Filament Admin Panel installed in your application.
-
A basic understanding of how Filament fields and forms work.
Problem Statement
When working with unique fields like email, you need to validate uniqueness for both creating and updating records. However, the validation logic needs to account for the current record being edited to avoid false positive errors.
For example:
-
Creating a Record: The email field must not already exist in the database.
-
Editing a Record: The email field must not already exist for other records but can remain the same for the current record.
Solution: Using unique()
with Filament's TextInput
Filament's TextInput
provides a built-in unique()
method that simplifies handling unique validation. Here's how to use it.
Code Example
Below is an example of how to make the email
field unique:
use Filament\Forms\Components\TextInput;
TextInput::make('email')
->email()
->required()
->unique(ignoreRecord: true),
Explanation
-
email()
: Ensures the input follows a valid email format. -
required()
: Makes the field mandatory. -
unique(ignoreRecord: true)
:-
This is the key to solving the problem.
-
The
unique()
method ensures that the field value is unique across all records in the database. -
Setting
ignoreRecord: true
allows the validation to ignore the current record being edited, avoiding unnecessary validation errors.
-
Where to Place This Code
This code should be added in the form schema of your Filament Resource. For example:
use Filament\Resources\Forms\Form;
use Filament\Forms\Components\TextInput;
class UserResource extends Resource
{
public static function form(Form $form): Form
{
return $form
->schema([
TextInput::make('email')
->email()
->required()
->unique(ignoreRecord: true),
]);
}
}
Testing the Implementation
-
Create a Record:
-
Try creating a new record with a unique email. It should succeed.
-
Attempt to create another record with the same email. Validation should fail.
-
-
Edit a Record:
-
Edit an existing record, keeping the email the same. Validation should succeed.
-
Attempt to change the email to one that already exists in another record. Validation should fail.
-
Conclusion
By leveraging Filament's unique()
method with the ignoreRecord
parameter, you can efficiently handle unique validation for input fields during both creation and editing. This approach ensures a smooth user experience and robust data integrity in your Laravel 11 application.
Feel free to customize the validation logic further as needed to suit your application's requirements. Happy coding!
Tentang Penulis
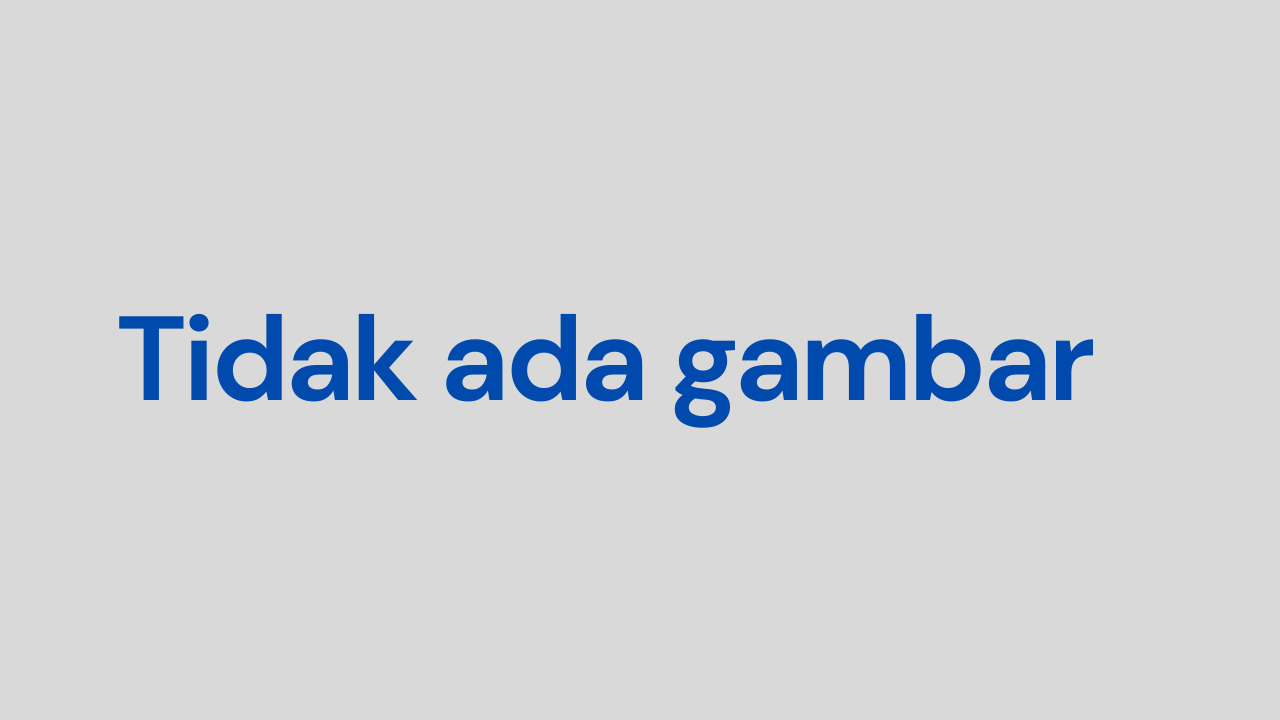
Muhammad Ilyas Mukhlisin
@ilyasmukhlisin